How To Create A Mobile App Using Android Studio
From games to scheduling their lives people use their phones and the applications contained therein for all manner of activities. One of the most powerful tools available to the developers of such applications is the ability to dynamically load and fetch pages and information from the web. This guide will walk through how to setup Android Studio as well as how to construct a simple web browser from scratch which can load web pages, refresh, and use a simple bookmarks menu. This guide will learn your the basics of creating android apps and is a great starting point before getting your hands dirty on more advanced Android App Templates available on Codester.
Installing Android Studio
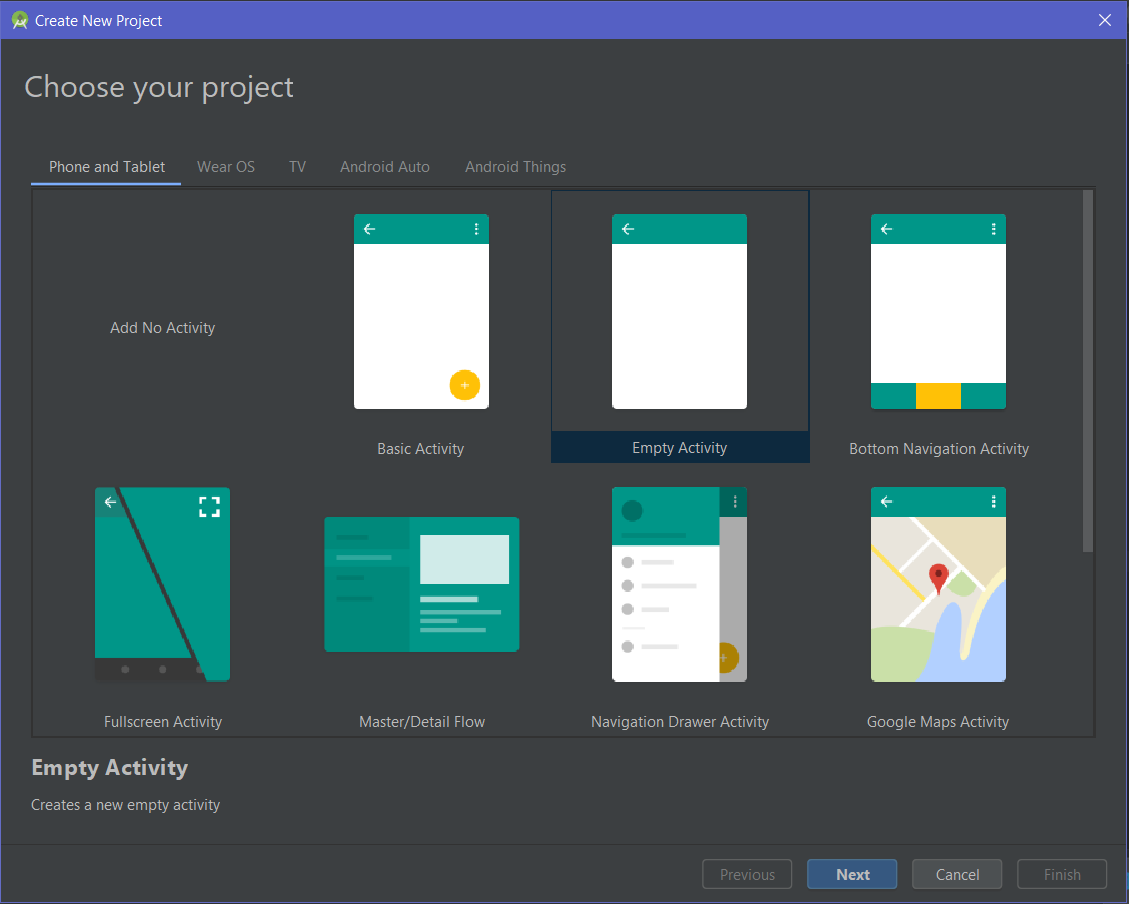
First things first, installing Android Studio is a simple affair. Using the following link: [https://developer.android.com/studio] select “Download Android Studio”. Then choose the location to be installed to making sure that it has a good amount of space because every application that is created within it as well as the Android device emulators that will be explained toward the end of the guide will be stored there.
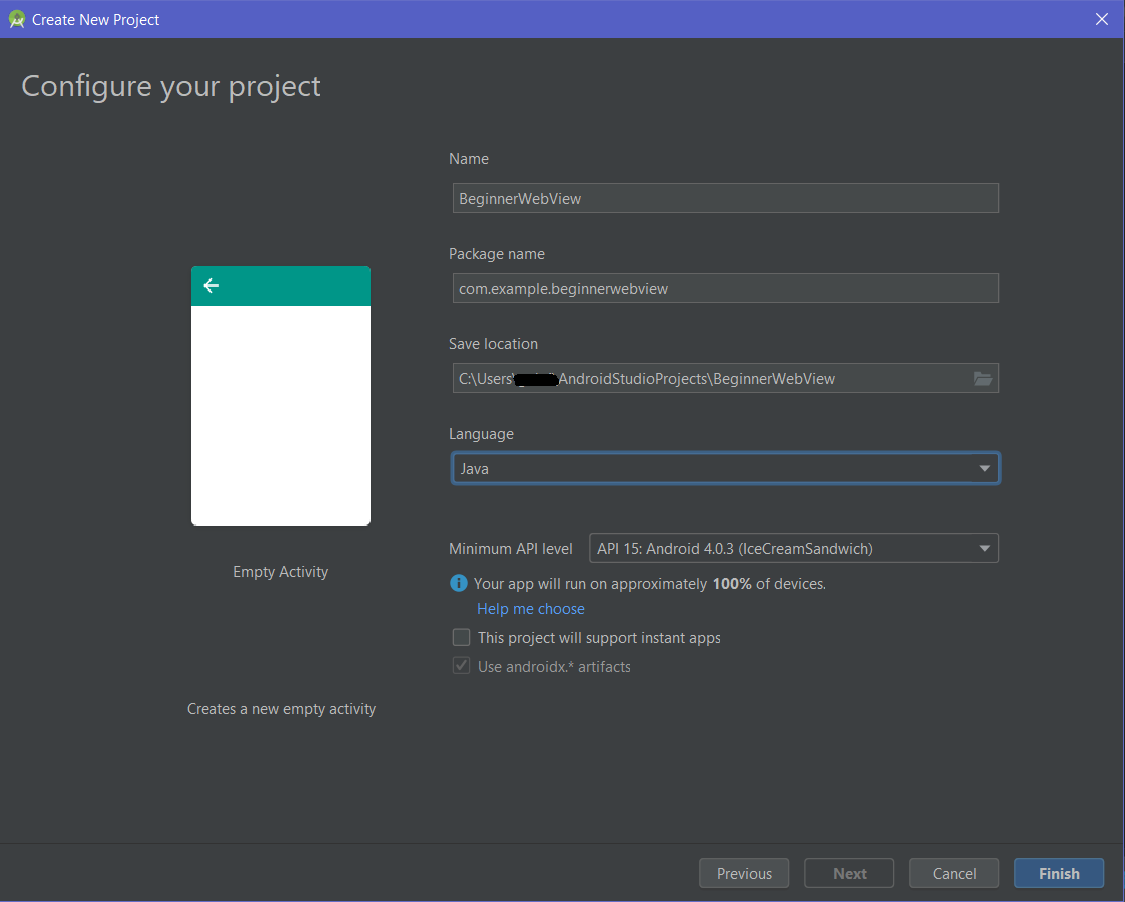
Once Android Studio is installed and opened you should see a welcome message prompting you to create a new project. Go ahead and accept that offer and then select to create an empty activity and then populate with a title and a location. Make sure to select Java when prompted to select a Language and we will be using API 15: Android 4.0.3 (IceCreamSandwich) for our minimum API level as demonstrated in the images. Then select Finish.
Developing the WebView Application
Now that Android Studio is up and running you should find yourself on the landing page full of menus and tiny icons and other intimidating features. Do not worry, we will get through this. Remember that even when the landing page is open Android Studio may still be building a few required files which may change the layout of the sidebar view, so to avoid having items jump around while you are trying to work with them, give the application a few minutes before moving on to the next step.
Step 1 – Layout
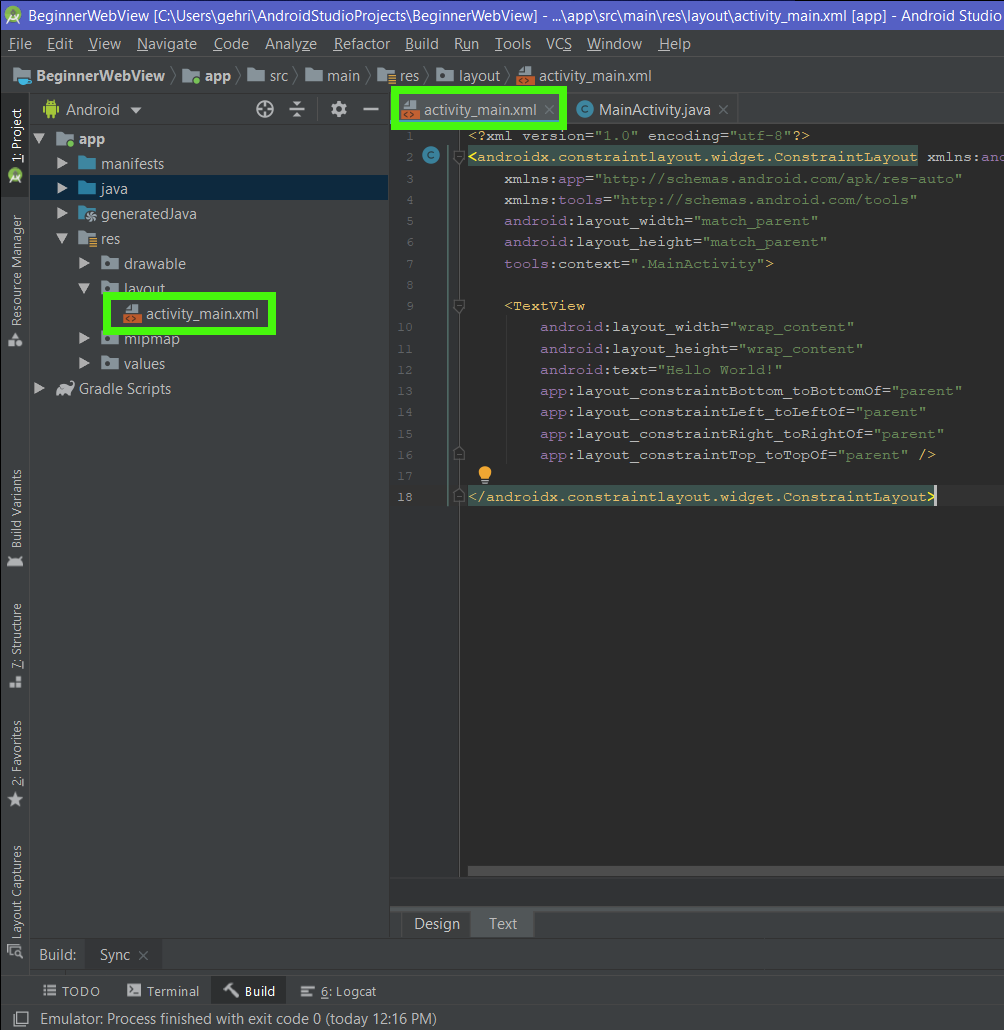
The first thing that needs to be done is to get the layout of the application to be correct, so navigate to the ‘activity_main.xml’. It should be along the top bar by default, however if it is not it can be found by navigating via the sidebar from “app > res > layout > activity_main.xml” as demonstrated by the image on the right.
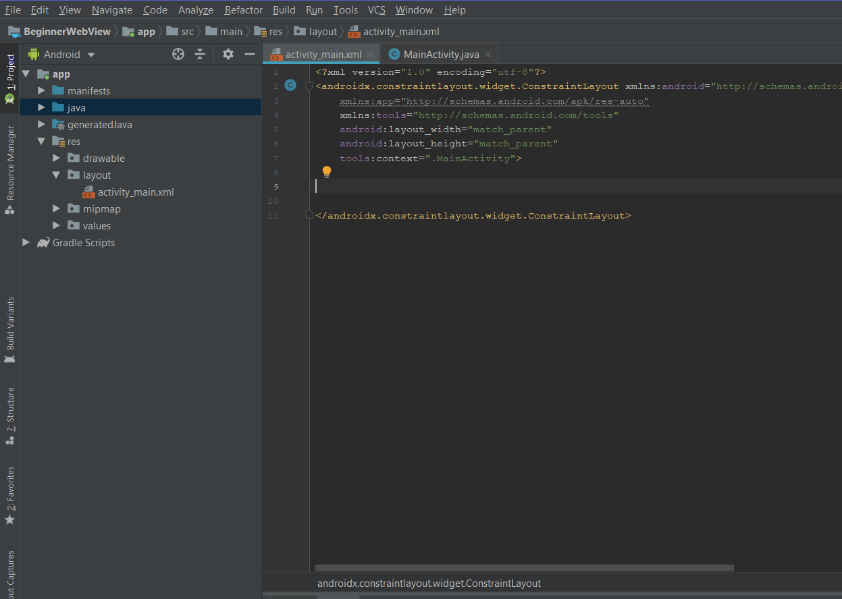
What I want you to notice on this page is the layout of the xml file. The gold text defines a Constrained Layout which contains the entire application. After the initial settings are defined for the Constrained Layout there is a TextView inside of it which then has a whole series of its own settings, including ‘android:text=”Hello World!”’ which is what controls what text is displayed on the page of the current application. Though TextViews are very useful tools what we need to do at this stage is to erase everything relating to the text view. On the example image that’s lines 9 -16. So that the text resembles the image here.
In the space left behind by the TextView we are going to create two nested Linear Layouts. A Linear Layout is a container which organizes its contents either horizontally or vertically one next to the other in a linear fashion. In order to create one, click on line 9 like in the image. Then press tab one time to create an indent and type “<LinearLayout” Android Studio will try to autocomplete the section for you. Once you type “<LinearLayout” just press enter and the section will be created for you. It will populate your layout with two settings, “layout_width” and “layout_height”. For both of these type in “match_parent”. After the “layout_height” setting there should be the following text “></LinearLayout>”. This indicates the end of the element you just created. So place your cursor between the “>” and “<” and press enter to expand out the layout so that it can contain element within it. Within this section press tab one more time for an additional level of indentation and create another Linear Layout exactly like before except with “layout_height” set to “40dp”. After you finish your page should resemble the following image.
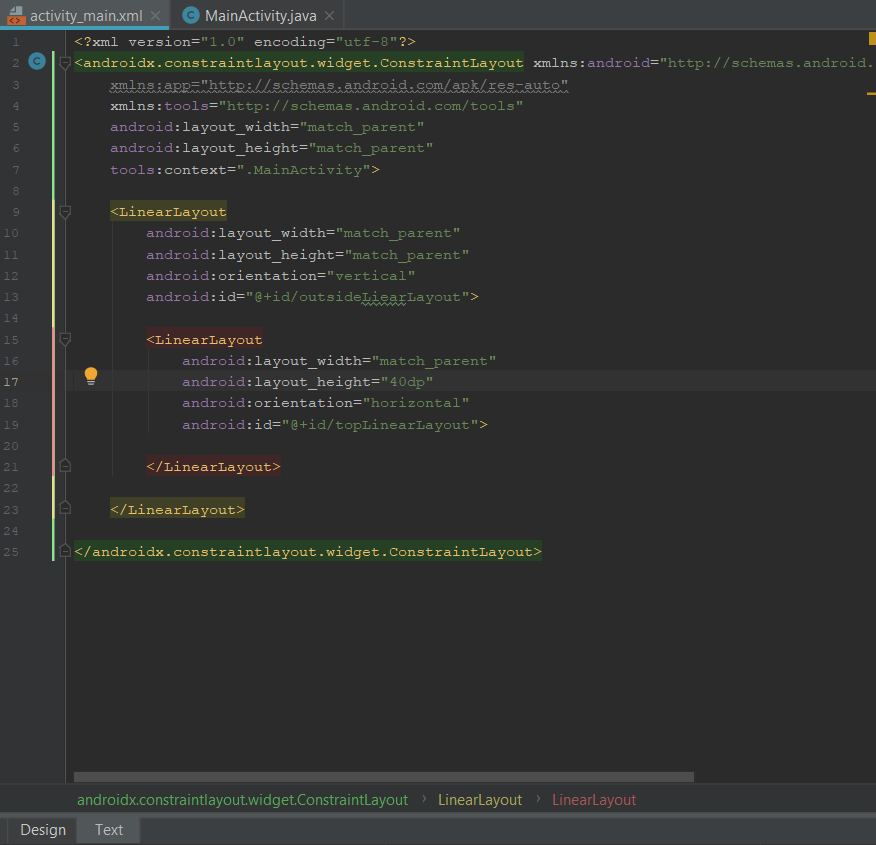
Once your Linear Layouts are set up we need to add a couple of settings to each of them. Starting with the first one you created, among the other android settings we need to see ‘android:orientation=”vertical”’. 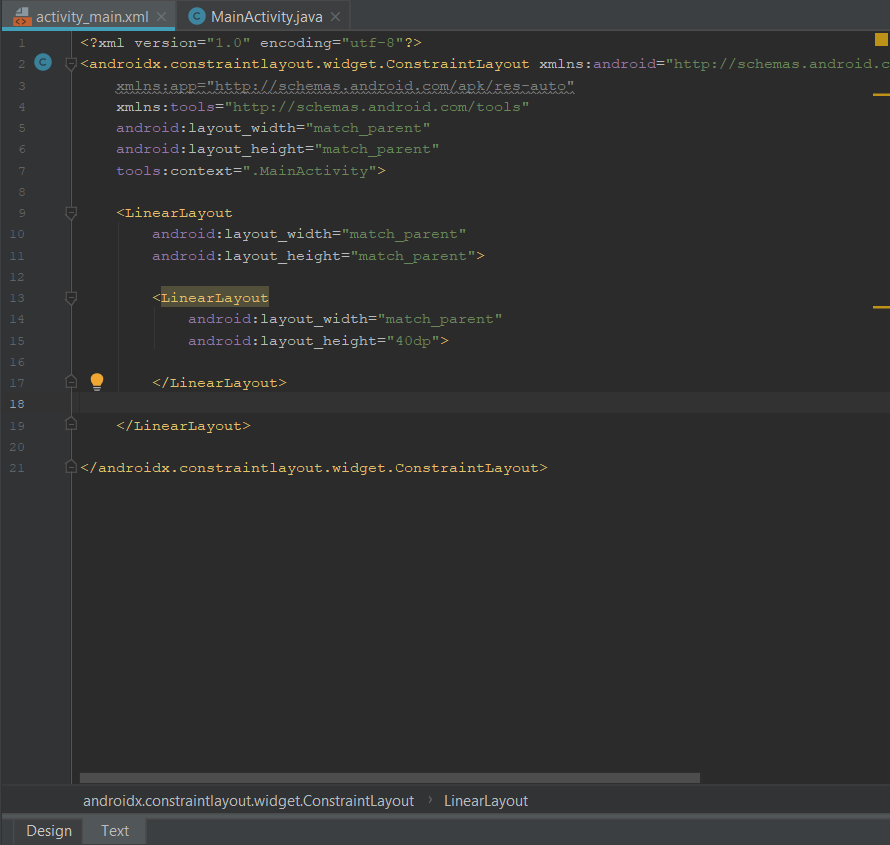
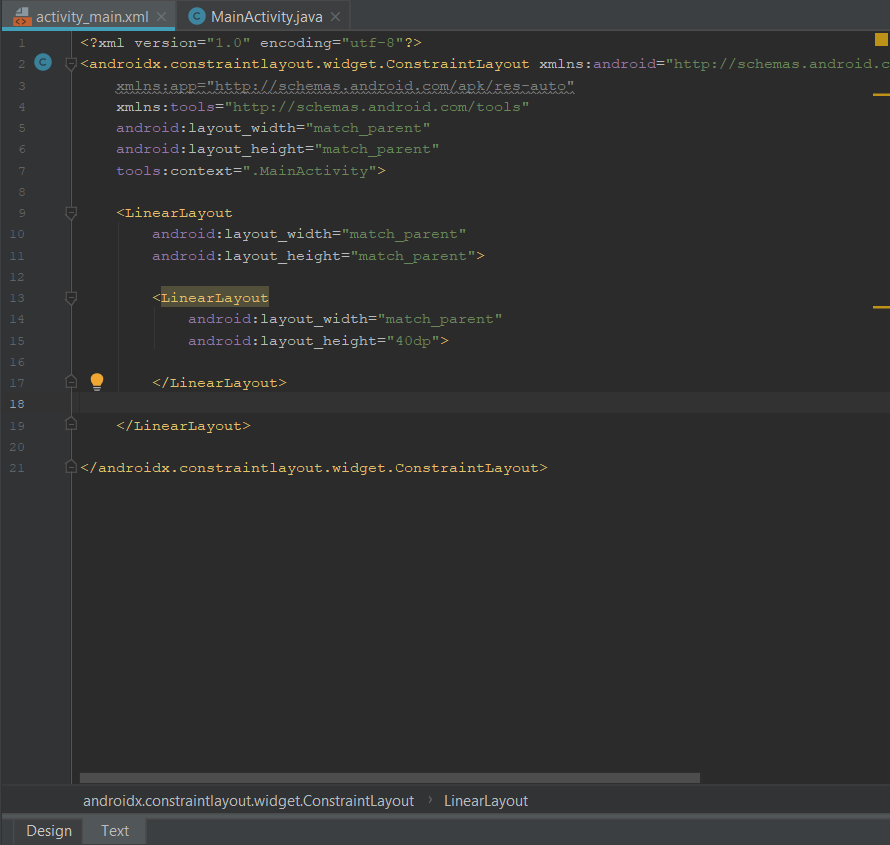
This controls the way the items inside of the Linear Layout are arranged. After that line, insert ‘android:id=”@+id/outsideLinearLayout”’ which will give the entire container the id of “outsideLinearLayout” for us to find from the Java code later on. The same will need to be done to the second layout with a couple differences. Firstly the orientation will need to be set to ‘horizontal’ instead of ‘vertical’ and the id will be ‘topLinearLayout’. [PS: Feel free to set the IDs to whatever you want, however if you follow what I am using it will be a bit easier to make sure your Java code is set up properly in the following step]
Now that’s it, your Linear Layouts are completely done, we will not be touching those again. Congratulations, but now comes the more interesting part. We are going to add another 3 elements to the xml file.
Image View: Contained within the second linear layout type “<ImageView” and press enter. Android Studio will automatically complete it like it did for the layout elements. For the layout_height and layout_width we once again want “match_parent” for both fields. Right under those settings we will be adding 3 more lines: ‘android:layout_weight=”0.9”’, ‘android:id=”@+id/iconView”’, ‘android:src=”@mipmap/ic_launcher”. The weight value will decide the importance of the image view. We want it to always be present and take as much space as it needs. This will be our icon preview of each page that the webview visits. The src value controls the default image that will be displayed in the image view, we are using a common image library and getting a simple android icon which will be replaced by any web page we visit.
Progress Bar: Right below the Image View we will type “<ProgressBar” and press enter. Again the sizes will both be set to “match_parent” and much like the image view we will add a few values to the settings: ‘android:layout_weight=”0.1”’, ‘android:id=”topProgressBar”’, ‘style=”@style/Widget.AppCompat.ProgessBar.Horizontal”’. The style one I’m sure looks a bit odd, but it is similar to getting the ‘src’ of the Image View. It looks through common Progress Bar styles and finds the horizontal styling that causes the bar to increase across the page as web pages are loading.
Web View: We made it, the reason you’re here. Below the second Linear Layout entirely (but still within the first) type “<WebView” and press enter. This one is actually fairly simple to set up on this page. 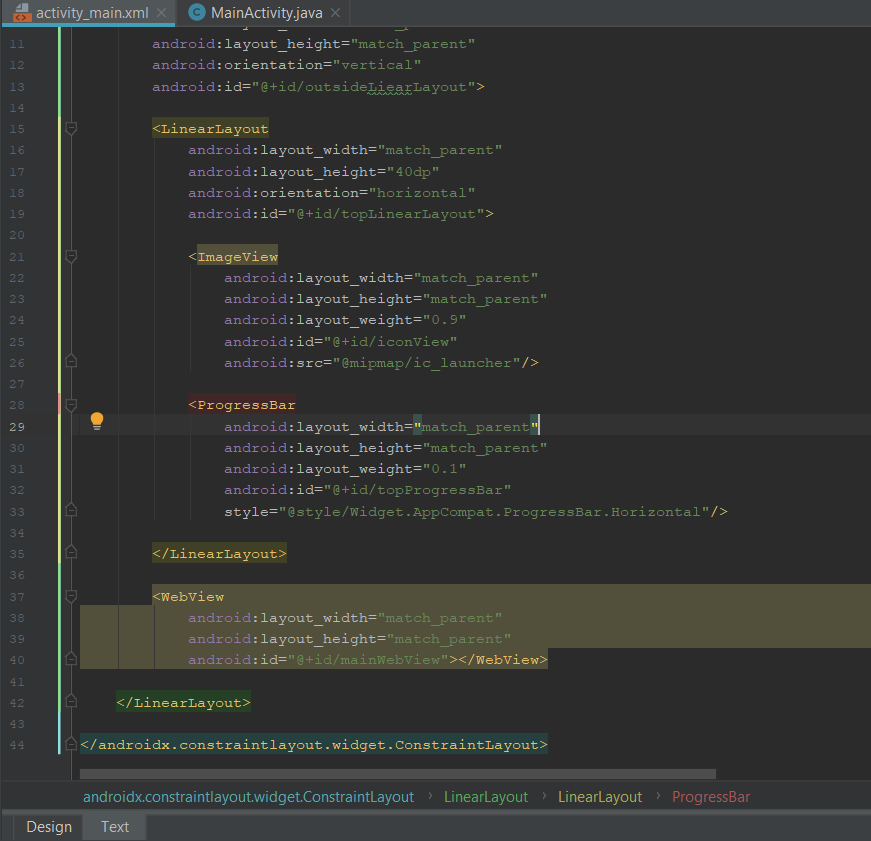
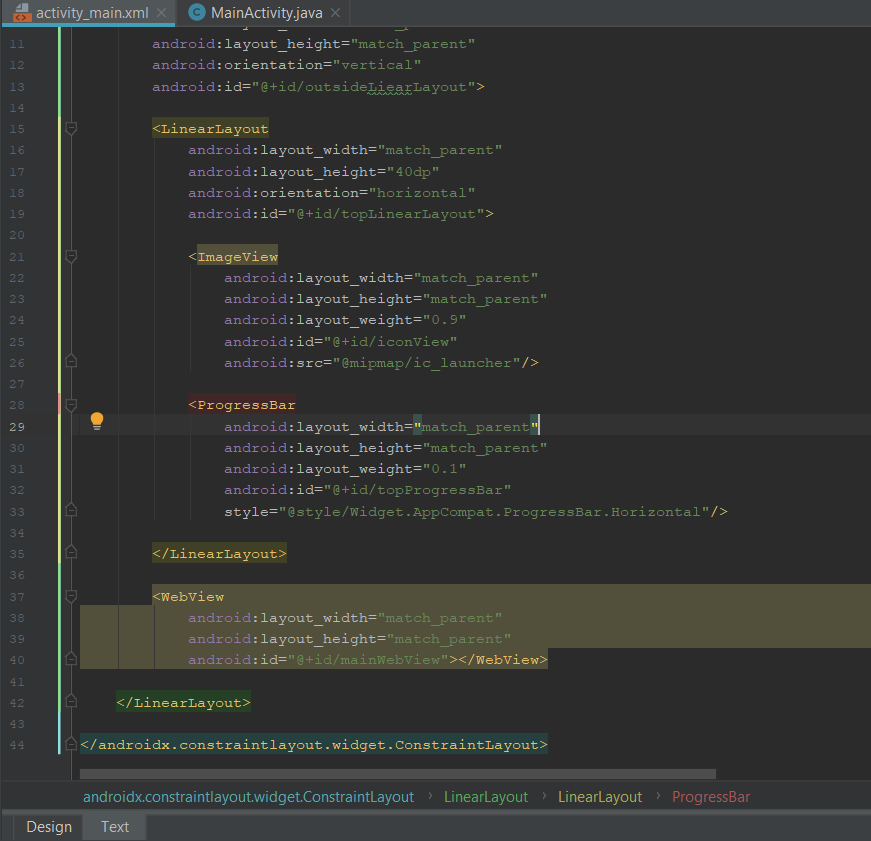
For height and width put “match_parent” and then create an id field like this: ‘android:id=”@+id/mainWebView”’ and believe it or not that is all we will have to do on this page.
I understand that those might have seemed like a lot but for reference your activity_main page should now look like the following image.
Step 2 – Java:
For now we are done with the layout elements. It is time to move from the ‘activity_main.xml’ file to the ‘MainActivity.java’ file. As seen in some of the previous images that should appear by default on the top bar next to the ‘activity_main’ file. If it does not, then the file can be found by navigating through the sidebar by going “app > java > com.example.beginnerwebview > MainActivity.java”. Keep in mind that ‘com.example.beginnerwebview’ is the name of the package that we set up at the beginning of the tutorial and this may be a different name for you.
In the MainActivity file we first need to establish a connection to the Layout elements we created in the xml file. In order to do that find the line that says ‘public class MainActivity extends AppCompatActivity’ and after this line create a section where we will establish the variables that will point to the elements in activity_main. Between the mentioned line and the first ‘@Override’ add the following:
LinearLayout outsideLinearLayout, topLinearLayout;
LinearLayout outsideLinearLayout, topLinearLayout;
ImageView iconView;
ProgressBar progressBar;
WebView mainWebView;
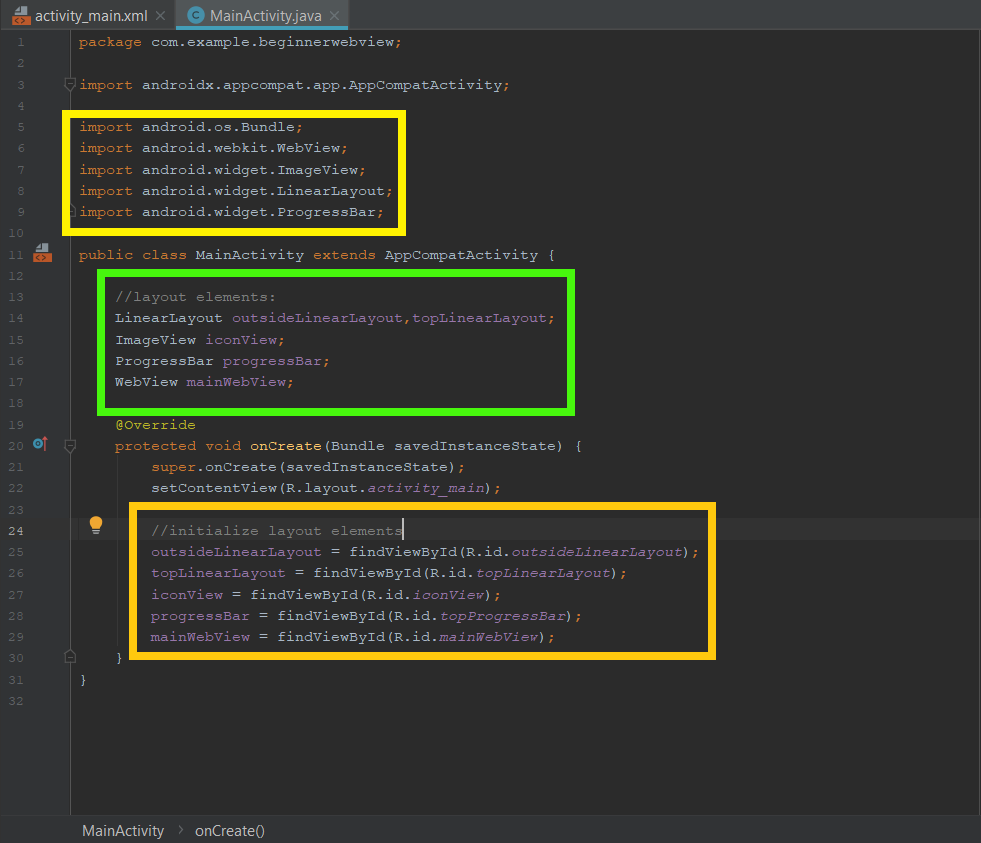
As you create each of these lines you should receive a prompt to import the associated libraries, just press enter to accept but if that is not offered the import statements will be highlighted in yellow in the left image.
To finish making pointers to our activity_main elements we will need to work within the ‘onCreate’ method. After the line ‘setContentView(R.layout.activity_main);’ add the following to finish pointed (highlighted in orange in the image):
outsideLinearLayout = findViewById(R.id.outsideLinearLayout);
topLinearLayout = findViewById(R.id.topLinearLayout);
iconView = findViewById(R.id.iconView);
progressBar = findViewById(R.id.topProgressBar);
mainWebView = findViewById(R.id.mainWebView);
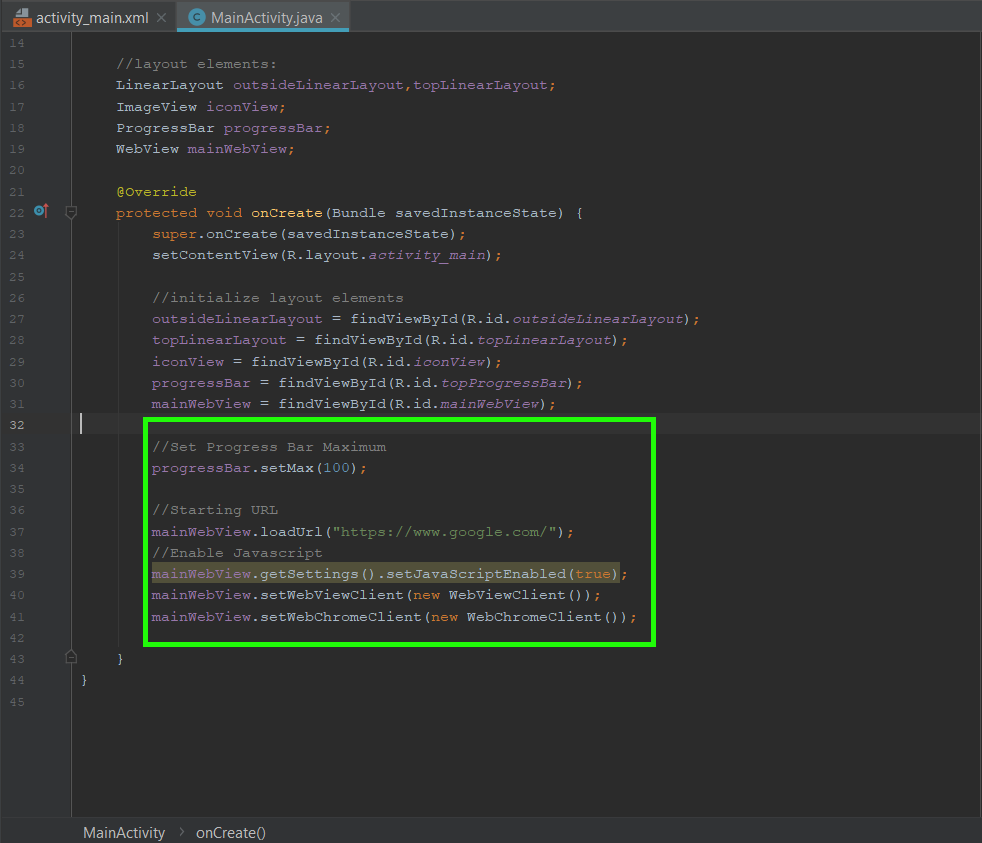
Keep in mind that the values that follow ‘R.id.’ are the names that we gave to the layout elements back in activity_main.
That being done, we need to set up some initial settings for our web view for it to work properly. So still within the onCreate method but below the other lines we added we will add a few lines of code to get things initialized properly:
progressBar.setMax(100);
mainWebView.loadUrl(https://www.google.com/);
mainWebView.getSettings().setJavaScriptEnabled(true);
mainWebView.setWebViewClient(new WebViewClient());
mainWebView.setWebChromeClient(new WebChromeClient());
Feel free to set the .loadUrl value to whatever starting webpage you want, I am going with google.com for simplicity’s sake.
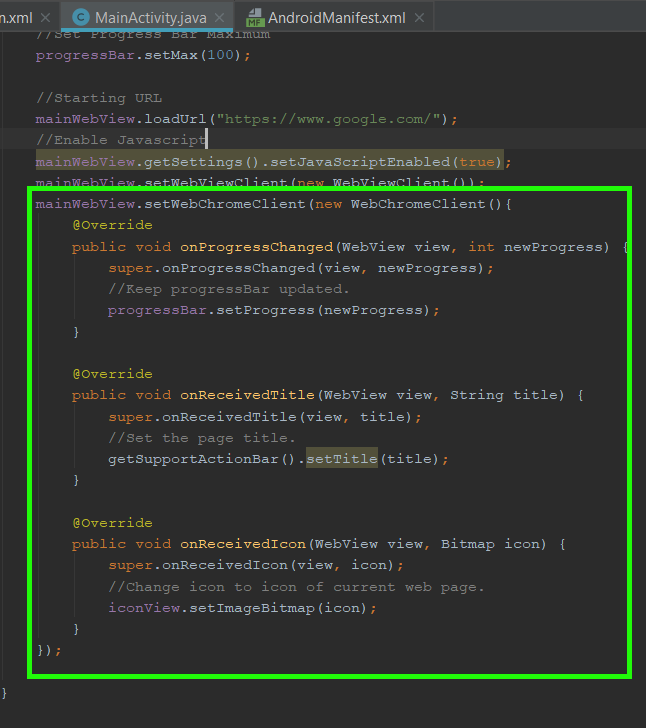
To finish this current batch of Java coding we need to edit the new WebChromeClient that you created to feed the required information to our Layout elements. So go back to the line reading ‘mainWebView.setWebChromeClient(new WebChromeClient());’ and right at the end between the final two parentheses (so between ‘()’ and ‘);’) create an open and closed curly bracket (‘{}’) and press enter to go to a new line between them. In this newly created section press ‘Ctrl+O’. This will open a new window called ‘Select Methods to Override/Implement’ we are going to select three of them:
‘onProgressChanged’
‘onReceivedTitle’
‘onReceivedIcon’
It does not matter in what order you select those three, but choose them all by Ctrl+clicking them and press ‘OK’. Now that those are created we are going to go through them one at a time and add in the required lines. Starting with ‘onProgressChanged’, inside of the method, just after the line that reads ‘super.onProgressChanged(view, newProgress);’ create a new line with the following text, ‘progressBar.setProgress(newProgress);’ This method will be called every time a page is loading and will dynamically update the level displayed by our progress bar. Now in ‘onReceivedIcon’ again as a new line at the bottom add the following, ‘iconView.setImageBitmap(icon);’. With this line in place every time the chrome client gets a new webpage it will provide the associated icon of that page to our iconView element. Finally under ‘onReceivedTitle’ in the same relative position as the other two add the line ‘getSupportActionBar().setTitle(title);’ This will change the title of our webviewer to the title of the current webpage as it loads.
And that is it for now. We will return to the java code, but for now give yourself a well deserved pat on the back, we have one more very small step and then we will have our first running demo.
Step 3 – Permissions and Demo
We need to find a new file on our sidebar again. Start by going to “app > manifests > AndroidManifest.xml”. This file controls the permissions that our application will be provided with while it runs. We are going to add a mere three lines to this page and then you can forget you ever saw it because that will be it. Find the ‘>’ on the third line of this page and place your cursor after it. Press enter to create a new open section between that line and the line that begins with ‘<application’. In this area we are going to enter the following:
<uses-permission android:name=”android.permission.INTERNET”/>
<uses-permission android:name=”android.permission.READ_EXTERNAL_STORAGE”/>
<uses-permission android:name=”android.permission.WRITE_EXTERNAL_STORAGE”/>

With those in place we now have all of the permissions we will need for the rest of this program completed.
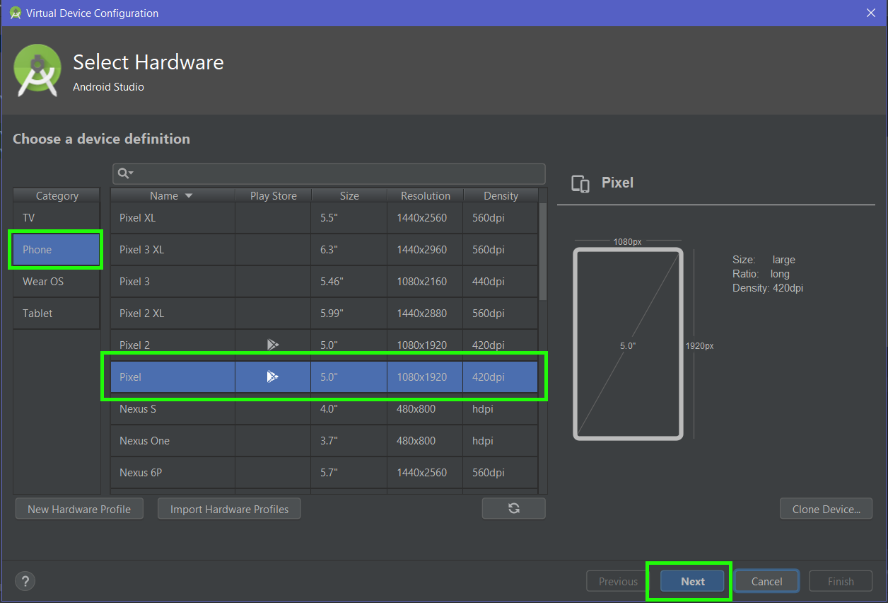
Now to see a demo of the application, click on the green play button in the upper right-hand corner of the window. This will open a new window titled ‘Select Deployment Target’. Your selection will likely be empty but we are going to fix that. Near the bottom of this new window click on the button titled ‘Create New Virtual Device’ which will open yet another new window (last one for sure) titled ‘Virtual Device Configuration’. Make sure that the category section on the left is set to ‘Phone’ and scroll to whatever android device you wish to emulate. I will be using a Pixel, but they should all work. Once you have it selected, click on Next.
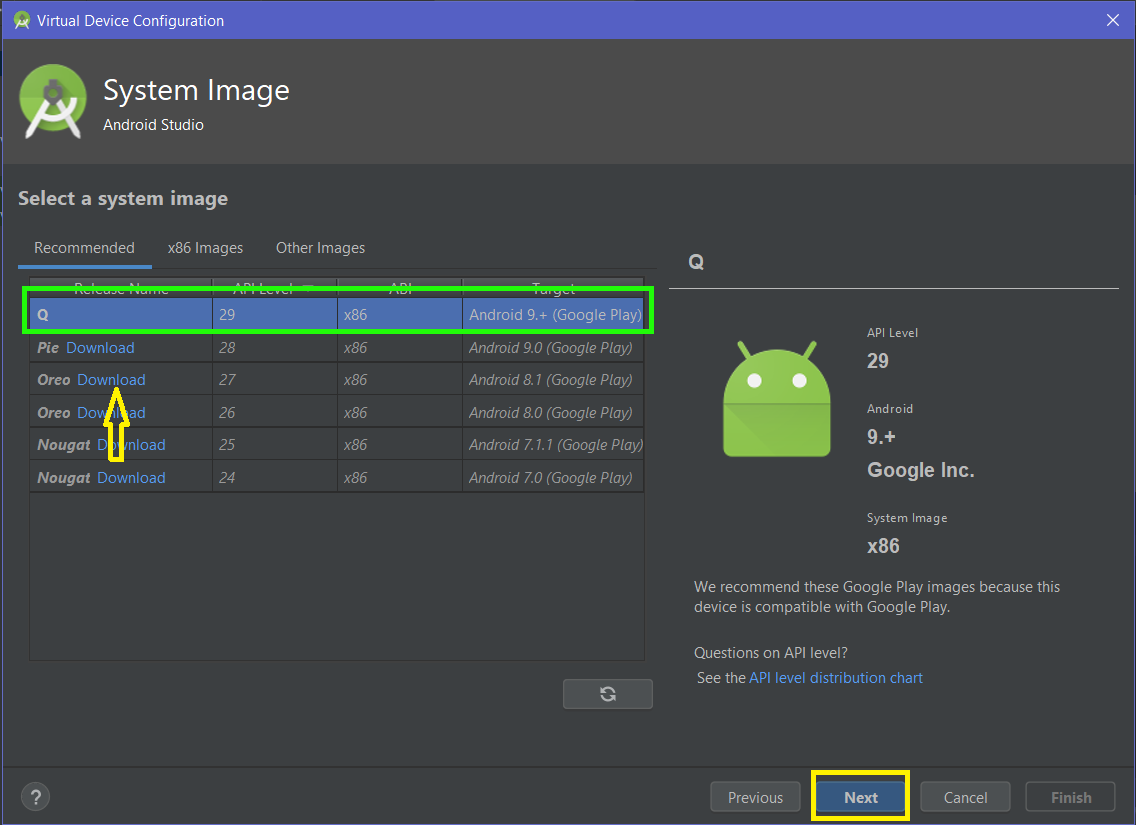
On the new page you are provided, you will be prompted to select a System Image. The specifics are not too important, but you will chose between a few different releases, I will proceed with Q because it is the most up to date at the time of writing. So make sure that you press ‘Download’ next to the name of the Release and wait for that to finish, then highlight the release and click on Next once more.
On the following page you will have the option to change the name of this Android Virtual Device (AVD), if you wish to. However this is not required. Simply click on Finish in the lower right-hand corner to proceed.
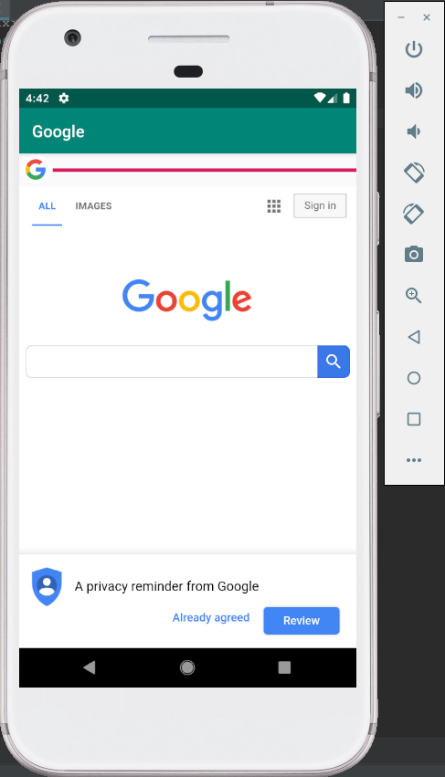
Now, back on the Select Deployment Target screen you should see the new virtual device available. Select it and click on OK. Give it some time to deploy as running an emulator of an entire new device can be rather processor intensive, and it will then have to install the new application you just made. After a couple minutes however you should see the phone screen with your new WebView application up and running showing either google.com or whatever web page you included as your landing page with the title of the page in the upper bar and to the left and the icon and progress bar located directly underneath. Congratulations. Because now we move on to the fun stuff.
Step 4 – Additional Functionality
So your WebView works. Great, but it is a little boring no? In this step we are going to add some functionality to what is already there. Starting with setting the progress bar to only display when the page is actually loading, after all we do not need to know that the page is at 100% all the time. Then we will enable refreshing the page when the user of the phone swipes up when the page is already scrolled to the top. Additionally if you were to press the back button at the moment the webview application would simply close, so we are going to add some functionality which will allow the application to go back to the previous page visited when back is pressed and only close the application once the user is back where they started and presses back again. Finally we may want to download something over the new browser at some point, or at least have the ability to, so we will set up a simple download manager.
Progress Bar Visibility
It is time to go back to the Java code. Remember how we expanded the new WebChromeClient by adding open and closed curly brackets? Because that’s what we are doing again for the WebViewClient one line above. Add in the open and closed brackets between the final two parentheses and expand. 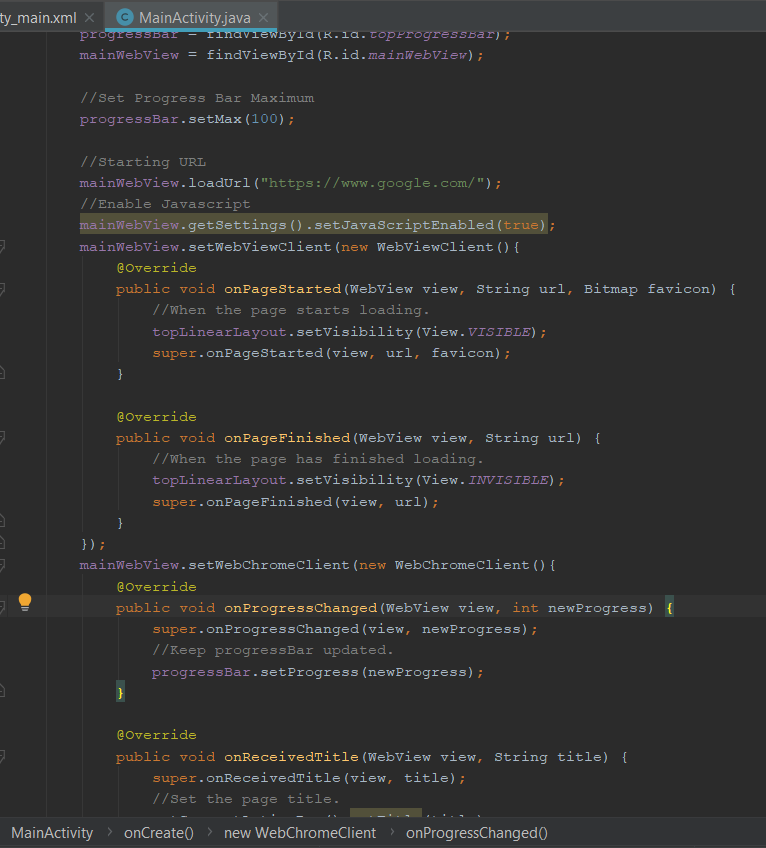
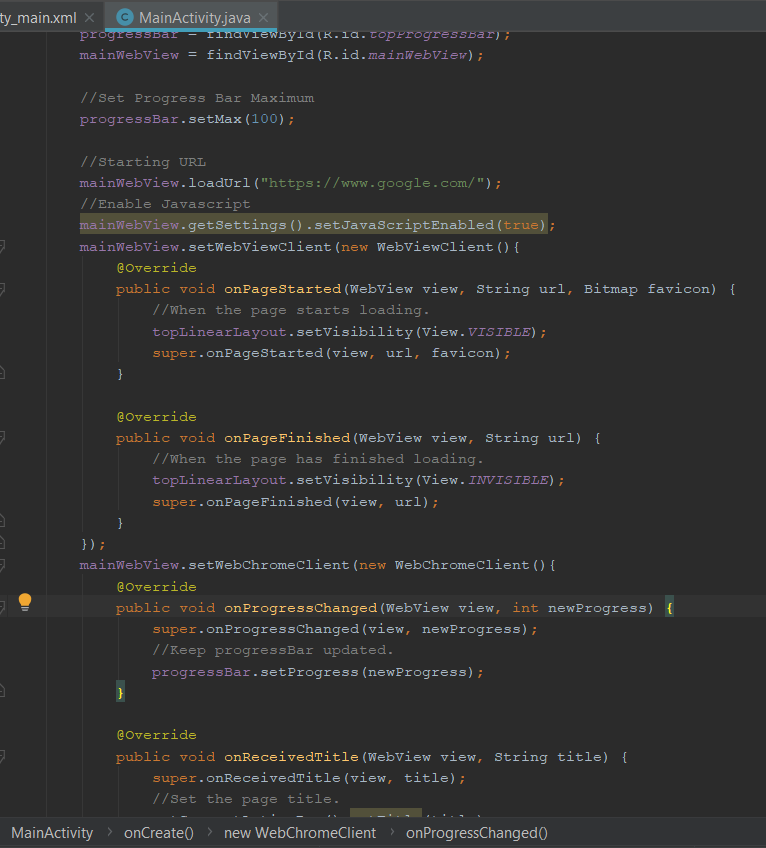
When you are in the new section that you made, press Ctrl-O again to open up the Select Methods to Override/Implement page. The two functions we are looking for are onPageStarted and onPageFinished. Select both and press ‘OK’. Of those two new functions, ‘onPageStarted’ is run whenever the page you are going to is currently loading and ‘onPageFinished’ is run when the loading has concluded. So starting with onPageStarted, put the following line: ‘topLinearLayout.setVisibility(view.VISIBLE);’
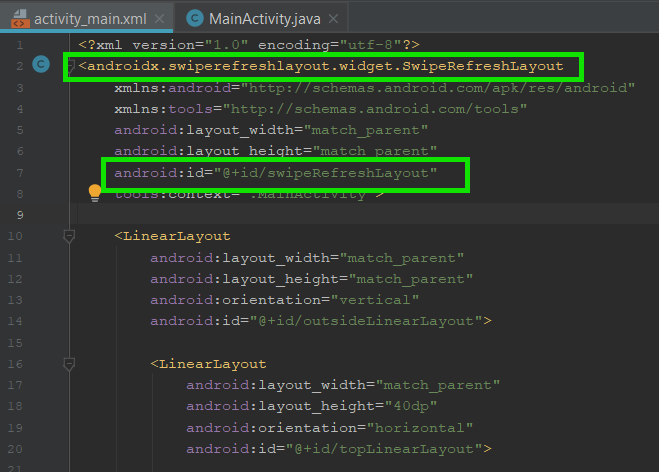
This will make the entire top bar of the page visible when the page is first fetched. Under onPageFinished put the exact same line except instead of view.VISIBLE put ‘view.INVISIBLE’ which will hide the top bar when the page has finished loading. What we will end up with is a rather cool visual effect where the bar drops down from the top of the page when a page is first requested, fills the loading bar as the page is loading and then neatly hides itself away after the page has finished loading.
SwipeRefresh
One feature you see very commonly on mobile browsers is the ability to scroll up even when the browser is at the top of the page in order to cause the page to refresh. It makes sense for us to implement that feature in our little application so let us go over how to do that. Starting with the activity_main.xml, this is where you created the general layout of the page. On the very top line we will be replacing the text that defines the Constrained Layout (that’s: ‘<androidx.constrainedlayout.widget.ConstrainedLayout’) with ‘<androidx.swiperefreshlayout.widget.SwipeRefreshLayout’. Note that it is important to only replace the text specified and no more. At the very bottom of the page now there should be a closing tag for the constrained layout ‘</androidx.constrainedlayout.widget.ConstrainedLayout>’ replace that as well with: ‘</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>’ to close the layout properly. Now to finish up with the .xml page, right under the open tag, we need to create an ID for the SwipeRefreshLayout. I will go with ‘android:id=”@+id/swipeRefreshLayout”’.
Unfortunately that does not quite take care of everything we need to do however we are done with ‘activity_main.xml’ for another good while. For now head on back to MainActivity.java. On this page we will be making a new variable pointer just like we did before for our layout elements only this one will be for a SwipeRefreshLayout. So near the very top of the page create a SwipeRefreshLayout variable (mine is named ‘swipeRefreshLayout’ because I’m uncreative). Then in the onCreate method point to the layout element using ‘findViewById’ just like we did for the other elements. The last thing we need to do to get the swipe refreshing working is to add a line in the java code ‘swipeRefreshLayout.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {});’ 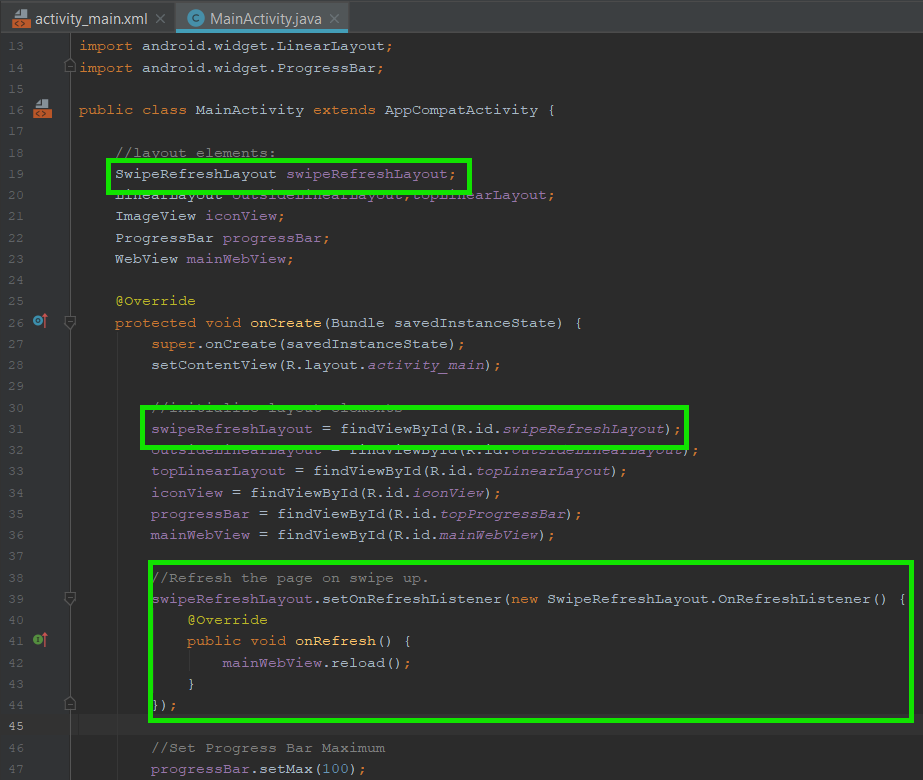
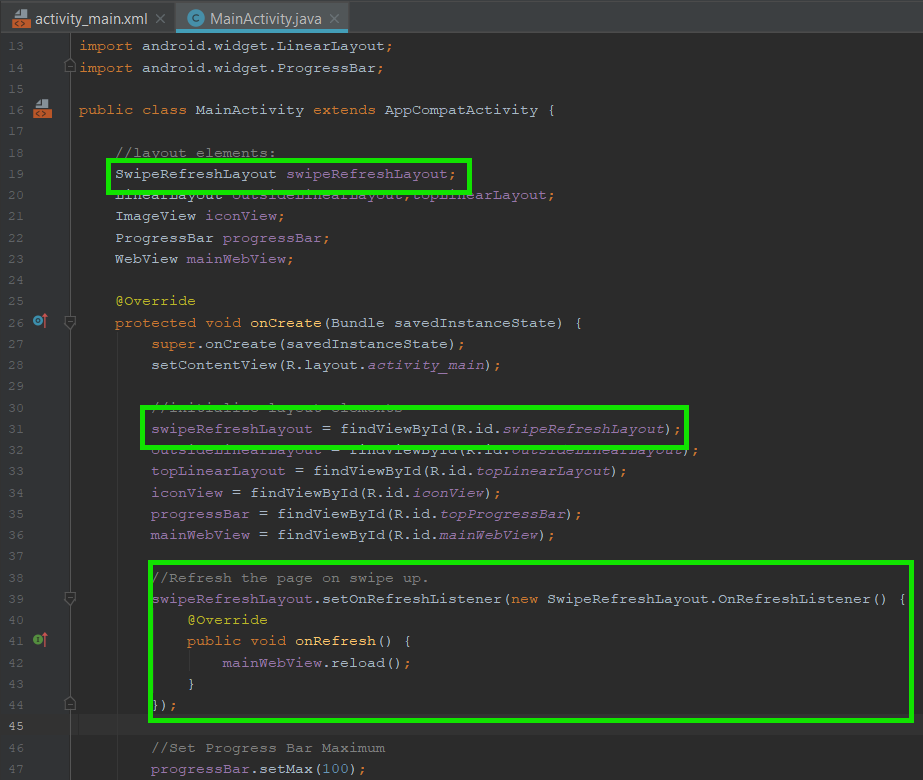
This should seem familiar from when we did the web view features. Anyway just like then put a couple new lines between the ‘{}’ and press Ctrl-O, on the Methods to Override/Implement screen we are looking for the method ‘onRefresh();’. In the onRefresh method that is created add the following line: ‘mainWebView.reload();’ and with that completed the screen should refresh when scrolling above the top of the webpage.
However, if you give it a try you will notice that once the page starts refreshing, the spinning wheel never goes away. Bit of an oversight of the function in my opinion, but we can fix it. You need to find the new WebViewClient we built before and go to the onPageFinished method. Go just beneath the ‘topLinearLayout.setVisibility’ method and enter the following line: ‘swipeRefreshLayout.setRefreshing(false);’. This will allow the spinning wheel to disappear after the page has been refreshed.
Back to go Back
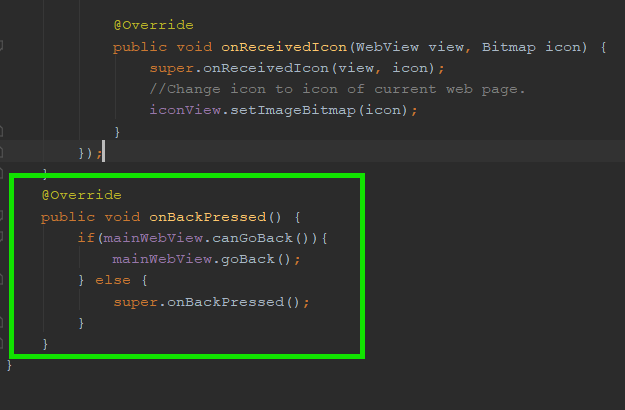
As mentioned above, at the moment if you were to fire up the application and press the ‘back’ button on the phone what would happen is that the application would completely close. This is not ideal as we would like to be able to use that to go to the previous page visited. Let us fix that.
Stay within the Java code and scroll down. Everything we have done so far has involved going into the onCreate method. This time however we will be going below that method (though still within the MainActivity). At the bottom of the java page there should be two ‘}’, the first one tabbed in once and the second against the left side of the window. After the first one, so between the two, create a new line and press Ctrl-O. The method we are looking for here is called ‘onBackPressed’ and is under the ‘androidx.fragment.app.FragmentActivity’ category. When you find it press ‘OK’. This method will be called every time the user presses the back button while the app is open. Currently the only line it contains is ‘super.onBackPressed();’ which basically says to just use the default functionality of the back button. We will be keeping that line but binding it in an ‘if..else..’ statement. So before that line type the following ‘if(mainWebView.canGoBack()) {‘ and press enter. Inside of the curly brackets enter ‘mainWebView.goBack();’. This tells the code that each time the back button is pressed, before closing the app first check if the web browser has pages in its history. If so, go back one page. In order to stop the app from closing when it recognizes that, go to the ‘}’ after the if statement and type ‘else {‘ and press enter. Put ‘super.onBackPressed();’ in this method. That will stop the code from executing the default functionality unless you are back to the first page of the browser.
Download Manager
Assuming that you may actually want to use the web browser to, you know, browse the web, then occasionally you are going to have to download something. Here we will quickly go over how to allow the webview application to handle a download request.
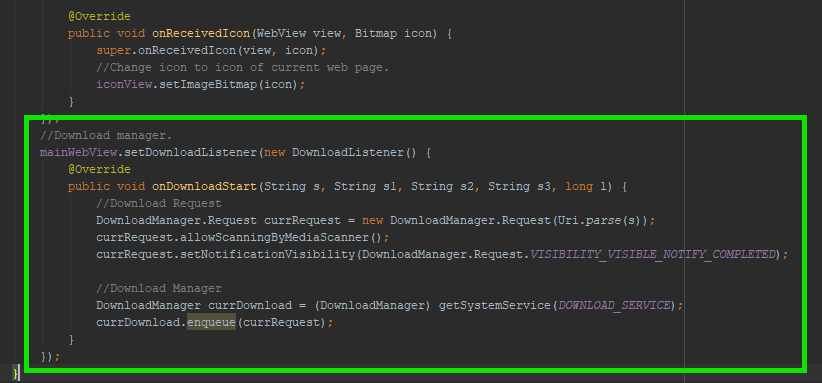
On the Java code go to near the bottom of the onCreate method where we created the new WebChromeClient. After that add a new line ‘mainWebView.setDownloadListener(new DownloadListener() {});’. We have been doing this for a while now, you know what is about to happen. After typing that line it should automatically open the curly brackets and add an override function called ‘onDownloadStart’ but if it does not, then open up the brackets yourself and press Ctrl+O and find the function in the window. Inside of the brackets for ‘onDownloadStart’ add the following line, ‘DownloadManager.Request currRequest = new DownloadManager.Request(Uri.parse(s));’. It is possible that ‘DownloadManager’ and ‘Uri’ will both show as red, this only means that the functions have not been imported. Click on them and press Alt+Enter to automatically import the required libraries. This will create an object we can manipulate containing the download request submitted by the web page. For our next line type in ‘currRequest.allowScanningByMediaScanner();’. This line is optional but ensures that when downloading media files like audio and video, the files will be listed in the native media player. Now that we have the download request and the ability to tell if it’s a media file, we need ‘currRequest.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED);’. Which will inform the download manager that we are to be notified when the download has finished. Now we have a request will all of the required settings done, we just need something to manage the request and get the actual file downloaded.
So, maybe leaving a bit of blank space to indicate that we are onto a new section, then put in the line ‘DownloadManager currDownload = (DownloadManager) getSystemService(DOWNLOAD_SERVICE);’. This will be our manager and be responsible for ensuring the file gets where it is going. So finally put in ‘currDownload.enqueue(currRequest);’. This is to pass our request to our manager which will start the download of the requested file.
Step 5 – Menu and Bookmarks
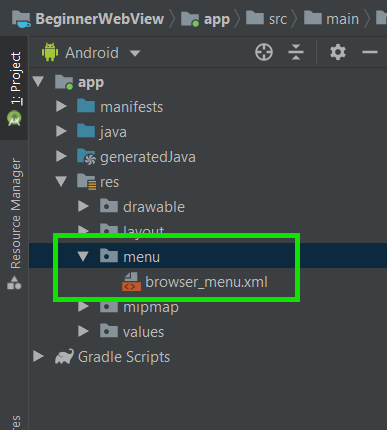
Now that we have ourselves a fairly well featured web browser, don’t you think that that top bar looks a little bare with only the web page title on it? Thought so. So for this step we will add a few icons to the menu bar as well as a short bookmark list. Go to the left column and find the following directory: app > res. Right click on the res folder and go to New > Android Resource Directory. This will open a window for the properties of the new folder. Change the default name to ‘menu’ as well as the type of the directory to a menu type in the dropdown underneath the naming bar. Now right click on this new directory you made and go to: New > Menu resource file. In this file the only parameter that needs setting is the name field. I will be using the name ‘browser_menu’ but feel free to use whatever you want and change the name in my code where appropriate.
You will likely recognize this file type as a layout file similar to the activity_main.xml we have been editing thus far. It will however only be used to edit the layout of the menu bar.
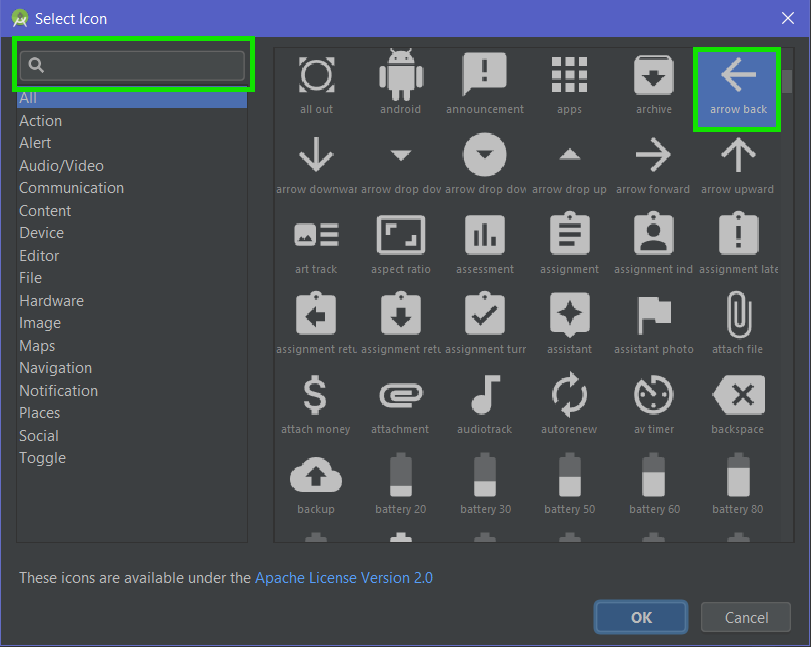
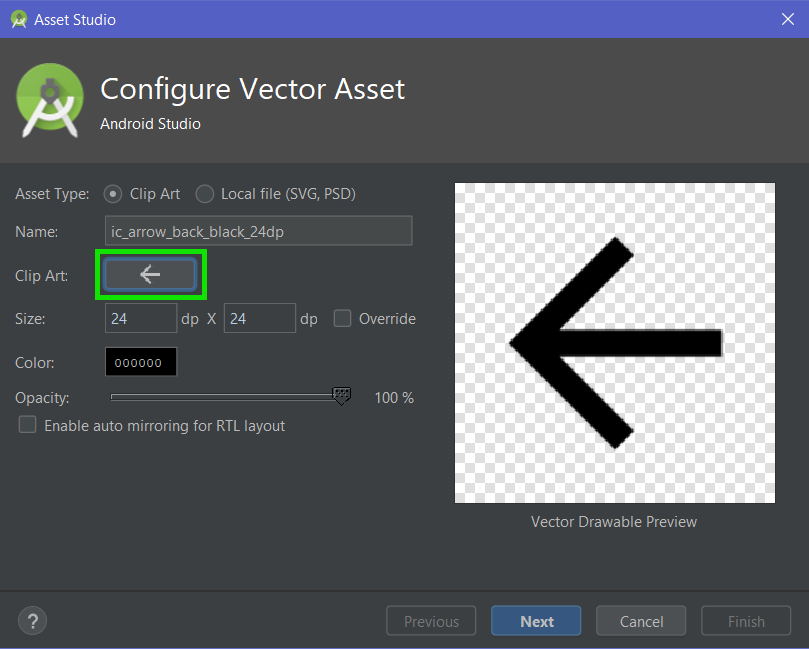
Before we continue with that however, open the ‘drawable’ directory in the same ‘res’ folder. Currently there should be two versions of the launcher icon we use for our Progress bar. We are going to want some buttons to press on our menu bar so we will be importing the requisite images here. So right click on the drawable folder and go to ‘New > Vector Asset’. This will open a new window specifically meant for selecting icons. On the left side of this new window there should be a section titled ‘Clip Art:’ and next to that title will be a small box with the current icon in it. Click on that box and after a second a new window will open up with a whole collection of icons available. We are going to grab a few from this window, starting with ‘arrow back’. There is a search bar in the upper left hand corner which can help with finding any of the icons I name. After you find the required icon click on ‘Next’ and then ‘Finish’ in the following page. Now go back and do it again for the following icons: ‘arrow forward’, ‘menu’, ‘refresh’.
Now that all of your icons are imported, click on ‘ic_arrow_back_black_24dp’ in the drawable folder to open up the .xml file to manipulate it. What you want to find should be on line 7, called ‘fillColor:’. Find the section in quotes that reads “#FF000000”. Change it to “#FFFFFFFF”. This is a hex code, we are changing the icon color from black to white which will make it much easier to see on the dark blue background. Now go through and do the same for all of the icons we just imported.
All right, as promised now go back to the browser_menu.xml file we created at the beginning of this section. We are going to be adding a bit of code to that now. Starting with the top line that contains the <menu declaration. Follow that line to the end and after ‘android”’ but before the ‘>’ insert a new line. The code to be entered here is ‘xmlns:app=”http://schemas.android.com/apk/res-auto”’. After that we can actually enter the menu and we will be inserting an ‘item’ element for each entry to our menu bar. Starting with the back button enter the following:
‘<item android:title=”Back”
android:id=”@+id/menuBack”
app:showAsAction=”always”
android:icon=”@drawable/ic_arrow_back_black_24dp”/>’
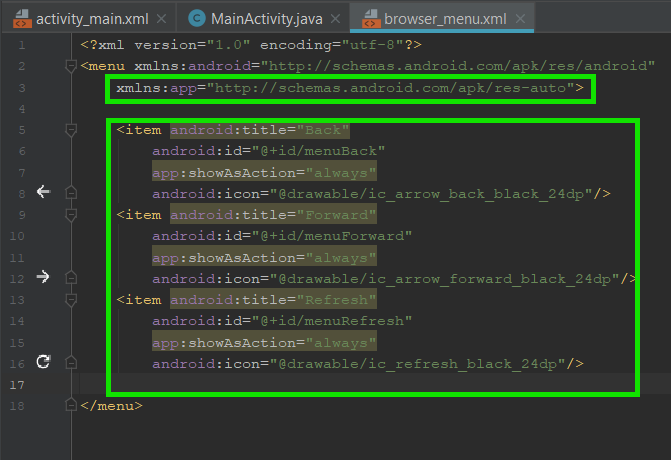
So let us go through what these items are. The ‘title’ is how the item will actually be saved in the menu bar, the id is something we have used before and will be used by us in the Java code to link to the button, showAsAction controls how often the button is in the menu and how often it is on the menu bar without needing to open the menu to see it, by setting it to “always” we are saying we always want it on the bar and not in the menu itself. Finally the icon element points to the icon we imported just previously which should currently be showing on your menu bar if you launch your application.
Now, following this same format, do the same for the ‘Forward’ button and the ‘Refresh button. The changes are ‘title=”Forward”’, id=”@+id/menuForward”, and icon pointing to the name of the icons we imported previously for this purpose.
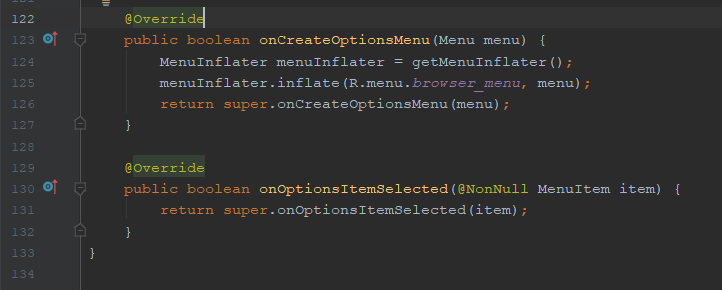
We will come back to this file briefly but for now go back to ‘MainActivity.java’. Find the area where we made the onBackPressed override method. Go after it and press Ctrl-O again. The methods we are looking for are titled ‘onCreateOptionsMenu’ and ‘onOptionsItemSelected’. Choose both of these and create the override methods. Go to ‘onCreateOptionsMenu’, in here we are going to be creating our own menu inflater. A menu inflater is an object that fills the options menu with the items available. So before the ‘return’ function add the following line: ‘MenuInflater menuInflater = getMenuInflater();’. And a variable is useless if you do not do anything with it, so then put : ‘menuInflater.inflate(R.menu.browser_menu, menu);’ This will tell the menu inflater to supply the option menu with our own custom made menu we just finished the layout for.
That will finish off that method. Now move down to the onOptionsItemsSelected method that we created right after it. In this method we will be receiving the menu item that was clicked on and telling the code what to do in response. We will start by creating a switch statement based on the MenuItem that is passed by default to the onOptionsItemSelected function. So between the ‘{‘ and the ‘return’ statement add the following ‘switch(item.getItemId()){‘ and press enter. This will allow us to switch between different methods depending on which item is selected. So start by entering a case item for each button we added to our menu so far. They can be added as follows:
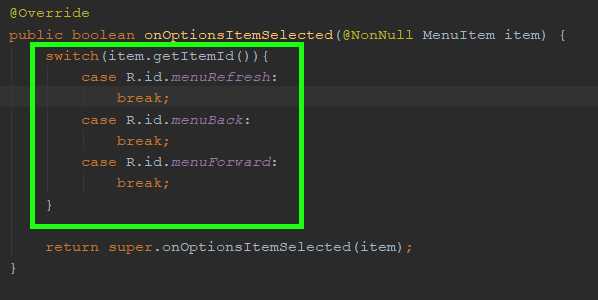
break;
case R.id.menuBack:
break;
case R.id.menuForward:
break;
Currently how the switch statement works is that when a menu item is selected the onOptionsItemSelected function is run with a parameter set to the button that was pressed. The switch statement then checks the ID of that button and checks to see if it has any cases set to that ID. Currently all the cases say to do is to break out of the switch statement but we will add some functionality here.
Starting with menuRefresh. If the user selects that button we want the page to reload the same as if the user scrolled up from the top of the page. Which as we experienced before is really just one simple line of code. So between the ‘menuRefresh:’ and the ‘break;’ add the line ‘mainWebView.reload();’
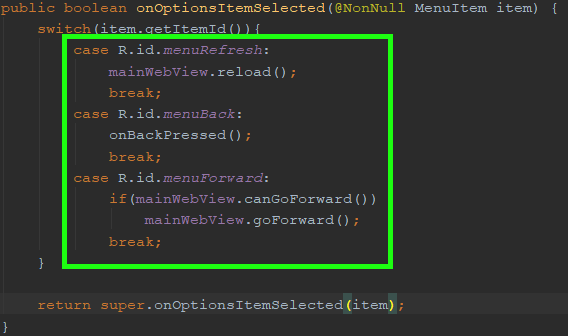
For the menuBack you might be tempted to do a similar chunk of code as we did for when the back button is pressed on the android device. And if you did it would work. However we do not need to spend that much effort. Between the ‘menuBack:’ and the ‘break;’ put the line ‘onBackPressed();’ to simply call the function above which can handle moving back through the pages all on its own.
Finally for the menuForward section. We will be adding some code in the same place. However because we do not already have a function built to handle going forward we will have to write a quick test to make sure there are no errors thrown. So in the same place add:
if(mainWebView.canGoForward())
mainWebView.goForward();
The ‘if’ statement will make sure we do not crash the app and only move forward if there is a forward element in the page settings.
At this point feel free to give it a try, all of the buttons we added to the menu bar should be working just fine.
Part 2:
Now we will add a small bookmarks menu with a few basic pages that any user of our webview might want to go to quickly. So to start on this we need to go back to ‘browser_menu.xml’. And back in this layout page we are going to add another 3 items (but feel free to add as many as you want) which correlate to different web pages. So just like we did before we will add these items in the format:
<item android:title=”XXX”
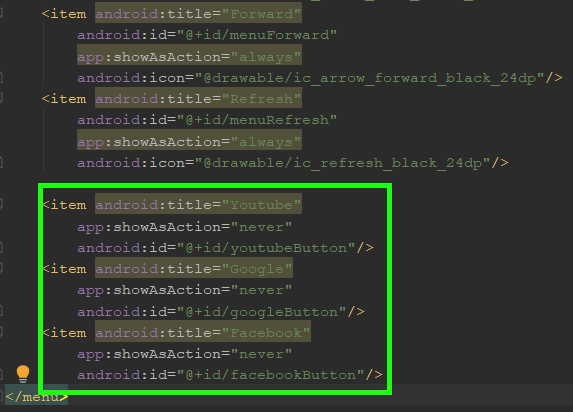
app:showAsAction=”xxx”
android:id=”@+id/XXX”/>
For these bookmarks options we want ‘app:showAsAction’ to equal “never” this is because the button will always be going in the bookmarks menu and is not to be displayed on the menu bar itself.
For my three webpages I am going to use “Youtube”, “Google”, and “Facebook” but feel free to point to whatever pages you want. To get there I am going to use the names of the pages as the titles (android:title=”Youtube”) and the name plus ‘button’ for the ids (android:id=”@+id/youtubeButton”).
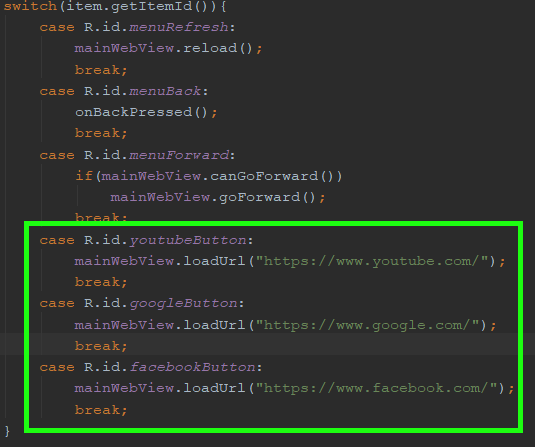
Now to get these buttons to work we will have to head back to the Java code and enter some cases for them inside of our earlier switch statement. So going back to that code inside of ‘onOptionsItemSelected()’ we will add a number of items to that switch statement equal to the number of pages that we wanted to add to the bookmarks bar. In this format:
case R.id.XXXbutton:
mainWebView.loadUrl(“https://>url here</”);
break;
With my versions demonstrated in the left image.
There is one final thing before we can call the bookmark bar done. If you were to open the android emulator now you would see three vertical buttons on the right side that when pressed open out bookmark menu. This works however I think it could look a little better. Also I had you create a menu icon way back at the beginning of this section so it would be a shame not to use it. Adding an icon for the menu button is not quite as easy as for the other buttons we put on the top bar because it exists already within the default layout for an android application. It can be done however. Start by going to ‘app > res > values > styles.xml’. This file contains information about the theme of the application and the top and bottom should be ‘<resource>’ tags with ‘style’ tags just inside of it. We are going to be adding an item to within the ‘style’ tags to start off. It will read
‘<item name=”android:actionOverflowButtonStyle”>@style/myActionButtonOverflow</item>’
Now that that is added with the other items, after the ‘</style> flag insert a new line. We are going to create our own style section. So type in:
<style name=”myActionButtonOverflow” parent=”android:style/Widget.Holo.Light.ActionButton.Overflow”>
</style>
This style element will now contain all of the display elements of the menu button. So, just within this new style section we will be adding two line to make the button look just like we want. The first should be similar to adding the icons to the top bar items:
<item name=”android:src”>@drawable/ic_menu_black_24dp</item>
<item name=”android:background”>?android:attr/actionBarItemBackground</item>
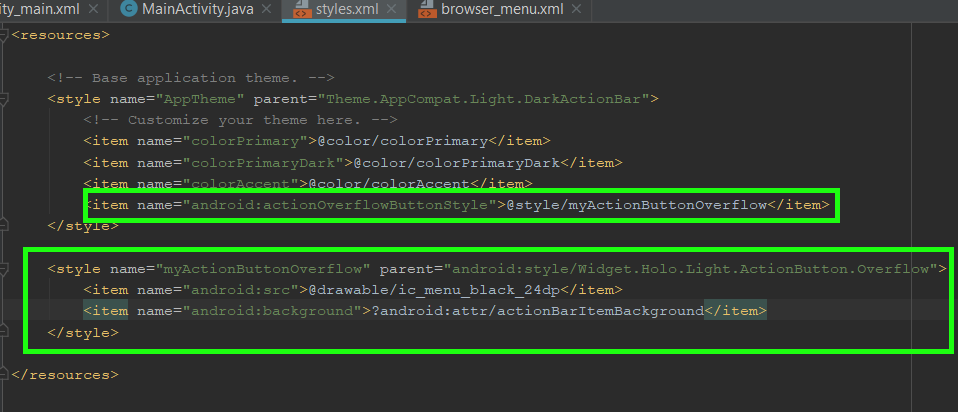
The first line there sets the icon to be the menu icon we set up at the beginning of this section. And the second line sets the background of the top bar to its default value. It is this line you will have to change if you want the top bar to be distinctly different. Finally the picture below shows what I ended up with after this whole process.
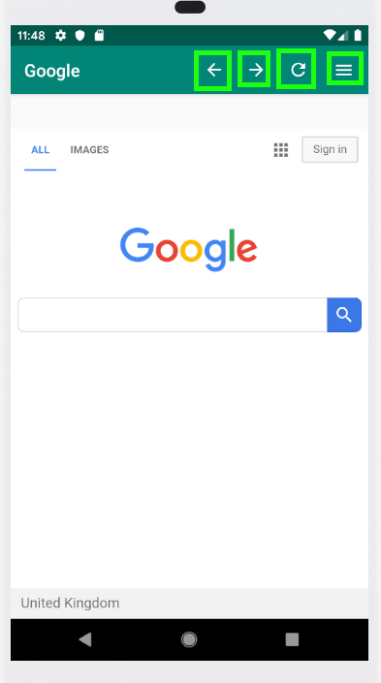
With that done our WebView application should be good to go. Fire up the emulator and give it a look.
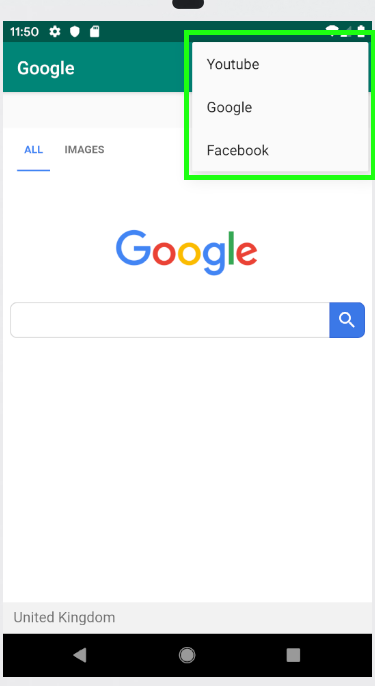
All four of our menu buttons should be set up with white on the dark green background. The pages should load just fine with the progress bar only visible while the page is actively loading. Finally the bookmarks button should open a small menu with the names of a few websites that when clicked on send the user to the site.
I hope you had fun learning a bit about Android Studio and WebView applications in particular, and see it is not so scary!
You can also download the full app source code here .
Once you are comfortable with the basics of Android Studio, there are many advanced Android App Templates available on Codester to kickstart you next mobile project.
Comments
Post a Comment
FOLLOW US ON FACEBOOK: https://www.facebook.com/unitechsity
:::. .:::
:::. .:::
Share or Like this Post - if you Find it Informative and Cool…
Thanks. Never miss any of my future post
CLICK HERE TO SUBSCRIBE
http://feedburner.google.com/fb/a/mailverify?uri=unitechsity
Once entered, you will have to check your inbox for a confirmation email containing a confirmation link. Once you VERIFY your email by clicking on the confirmation link in the message, you will never miss any future articles.
.